Build your new investment app
X times faster
than you thought.
Tradematic Cloud is a back-end infrastructure for building investment and trading apps.
LEARN MORE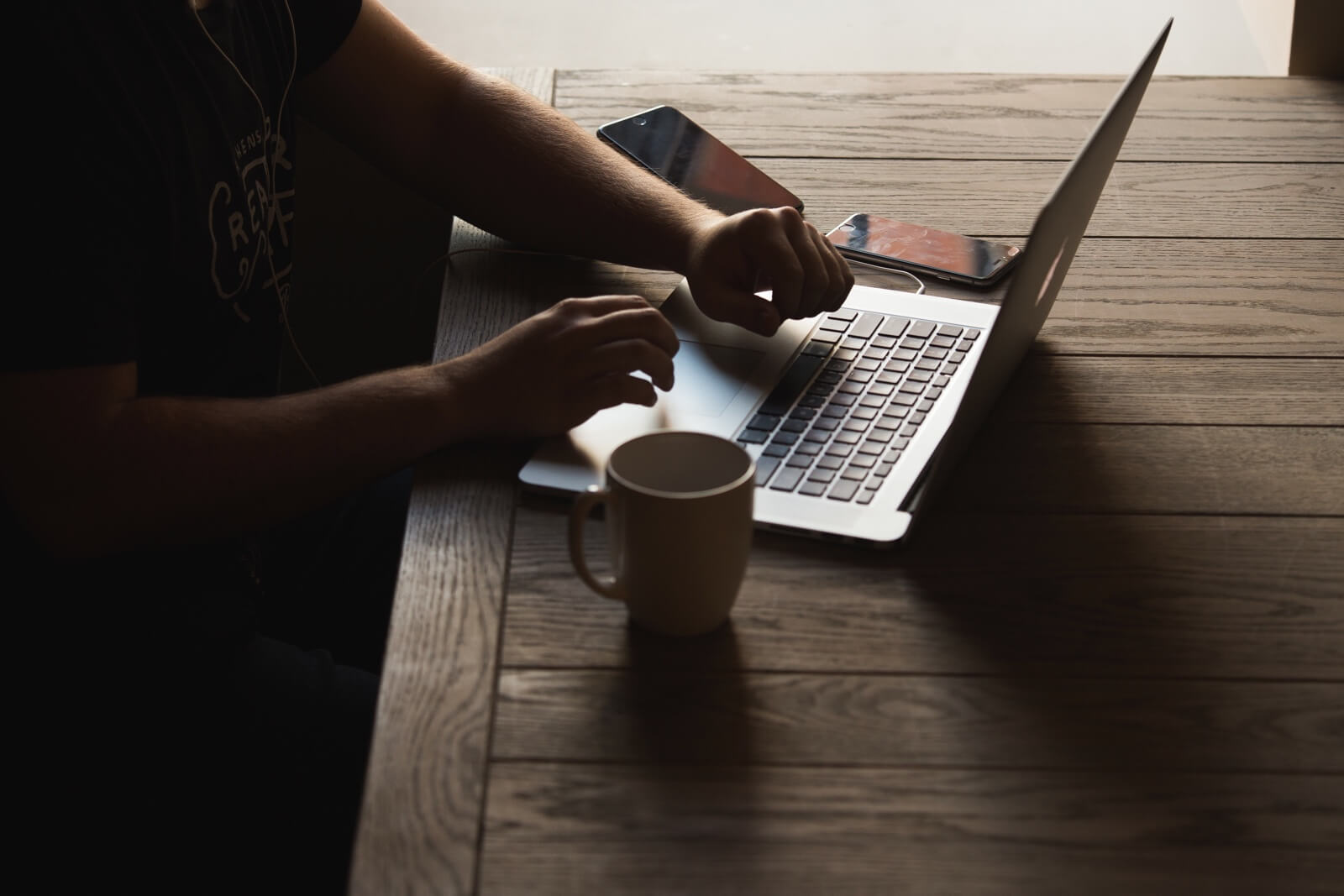
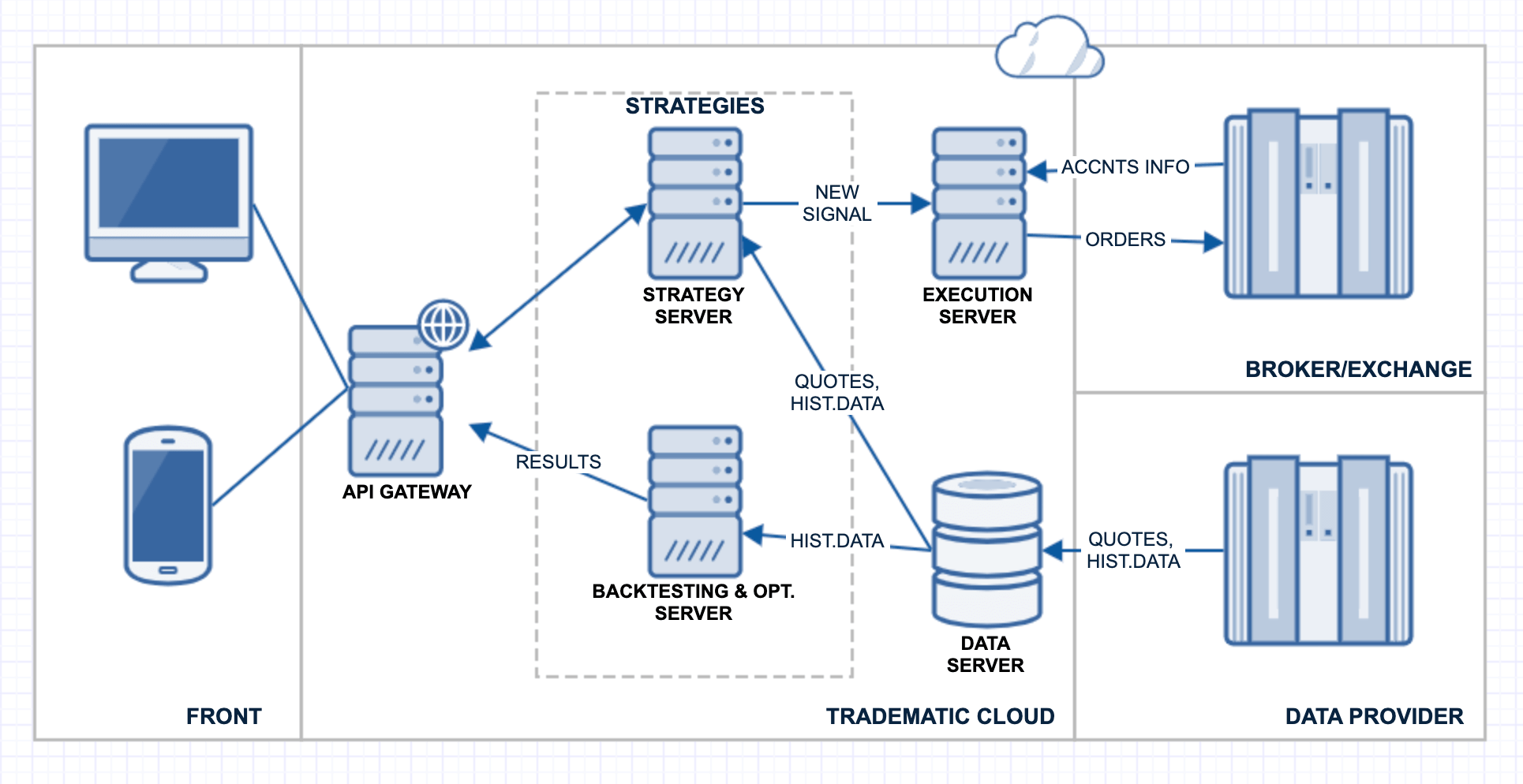
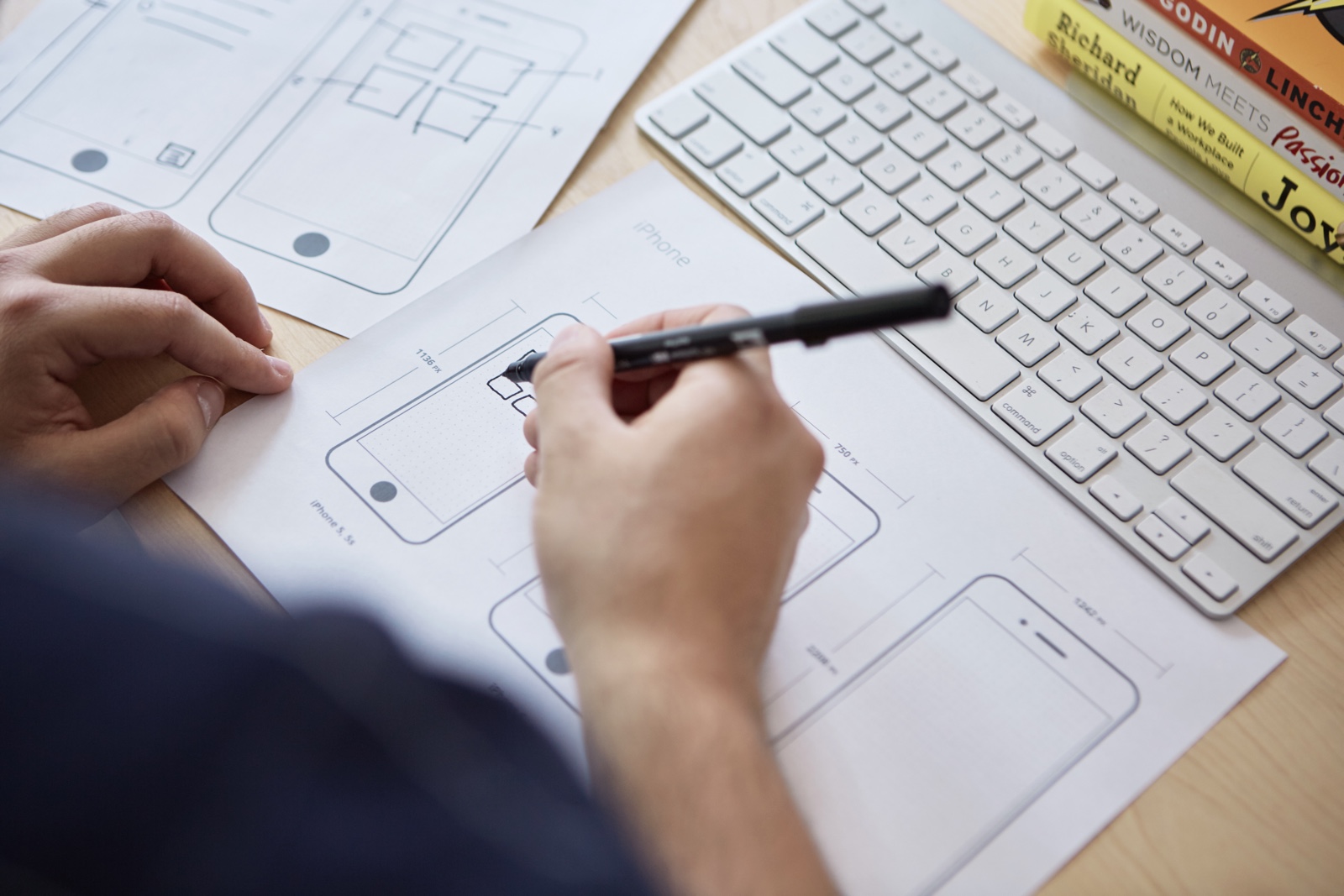
Developer?
Build your investment app using our powerful cloud API or deploy all the infrastructure on your side. All you need to do is customize your front-end.
You can start for free in our API Sandbox.
Busy?
We will help you to find experienced developers who have completed integration projects with our API.
You can start by choosing the right solution in Build with Tradematic Cloud section, or contact us to evaluate your project costs.
Tradematic Back-End Infrastructure
Core Features:
Portfolio management
Trading, accounts & positions
Multi-broker, multi-market, multi-account trading
Algorithmic trading
150+ technical and statistical indicators
Copy trading
Marketplace
Historical market data + real-time quotes
Backtesting & optimization
Server-side execution with order&risk management
Embedded referral programs
Notifications via SMS, email, messengers
Clients management automation
Online monitoring & analysis
Professional support
Adapters (Trading & Market Data):
FIX (universal)
Interactive Brokers (Stocks, ETFs)
Alpaca (Stocks)
Oanda (Forex)
Bitfinex (Cryptocurrencies)
Binance (Cryptocurrencies)
Poloniex (Cryptocurrencies)
Coinbase Pro (Cryptocurrencies)
HitBTC (Cryptocurrencies)
Kraken (Cryptocurrencies)
Tinkoff Investments (Stocks)
Market Data:
Alpha Vantage (Intraday and EOD)
Yahoo Finance (EOD)
Polygon (Intraday and EOD)
Build with Tradematic Cloud
Visual Strategy Builder + Automated Trading Platform
Investment Portfolios Marketplace
Client Asset Management
Risk Management System
Smart Currency Exchanger
Multi-Broker Account Analytics and Reporting
Chatbot with
Robo-Advising
Trading Ideas Execution on Social Media/Messengers
Multi-Broker Trading Platform
Live Quotes Widget
News-Based Trading
Web-Based Arbitrage Platform
Portfolio Management App
Copy Trading / Social Trading / PAMM
Educational Trading Desktop Terminal
Market Scanner
Voice Assistant as a Trading Interface
Your Special Case
Mobile Trading
Apps
ML-Based Trading Bot
Chatbot as a Trading Interface
Client Area
Plain-English Strategy Development Compiler
Tradematic API Samples
See how simple it is to use our API-first service.
-
Get trading account info & positions
import requests import json # Replace with the correct API key apikey = "YOUR_API_KEY" # Make a REST API call resp = requests.get("https://api.tradematic.com/cloud/accounts", headers = {"X-API-KEY":apikey}) # Get all trading accounts accounts = json.loads(resp.content) # Let's take the first one account = accounts[0] print("Account name: " + account["account"]) # Get account value and cash balance value = account["value"] cash = account["cash"] print("Value: {0} Cash: {1}".format(value, cash)) # Get account positions positions = account["positions"] # Let's show them for position in positions: print("Symbol: {0} Position size: {1}".format(position["symbol"], position["size"]))
-
Buy portfolio of stocks
import requests import json # Replace with the correct API key apikey = "YOUR_API_KEY" # Let's add Apple, Facebook, Microsoft, and 3M stocks to our portfolio portfolio = ["AAPL", "FB", "MSFT", "MMM"] # Let's take id of the first of trading account resp = requests.get("https://api.tradematic.com/cloud/accounts", headers = {"X-API-KEY":apikey}) accountid = json.loads(resp.content)[0]["accountid"] # Now buy the porfolio items, allocating 25% of the account value to each item for item in portfolio: order = { "order": { "symbol": item, "buy": "BUY", "shares": "25%", "price": 0, "type": "MARKET" } } resp = requests.post("https://api.tradematic.com/cloud/accounts/{0}/orders".format(accountid), data = json.dumps(order), headers = {"X-API-KEY":apikey})
-
Create strategy, based on SMA indicator
import requests import json # Replace with the correct API key apikey = "YOUR_API_KEY" # Let's find a rule for entry - price is above the SMA resp = requests.get("https://api.tradematic.com/builder/rules?filter={0}".format("price is above the sma"), headers = {"X-API-KEY":apikey}) entryRule = json.loads(resp.content)[0] # Let's find a rule for exit - stop-loss (%) resp = requests.get("https://api.tradematic.com/builder/rules?filter={0}".format("stop-loss (%)"), headers = {"X-API-KEY":apikey}) exitRule = json.loads(resp.content)[0] # Add parameters to the strategy strategy = { "strategy": { "strategytypeid": 4, # "4" for strategies that use ready-made rules for entry and exit "author": "me", "name": "My new SMA strategy", "description": "Description of my new strategy", "symbols": "MSFT,AAPL", "marketname": "NASDAQ", "timeframe": 15, "positionsize": "100000,50", # initial capital and 50% pos.size "content": { "rules": { "longentry": [ { "id": entryRule["guid"], "parameters": ["Close",9,"Red"] # SMA(Close, 9) } ], "longexit": [ { "id": exitRule["guid"], "parameters": [5] # stop-loss 5% } ] } } } } # Call the API method to create this strategy requests.post("https://api.tradematic.com/autofollow/strategies", data = json.dumps(strategy), headers = {"X-API-KEY":apikey})
-
Backtest strategy on historical data
import requests import json import time # Replace with the correct API key apikey = "YOUR_API_KEY" # Let's find strategy with name = "SMA-9" resp = requests.get("https://api.tradematic.com/autofollow/strategies?filter={0}".format("SMA-9"), headers = {"X-API-KEY":apikey}) strategy = json.loads(resp.content)[0] # Let's create a new backtesting task with our strategy task = { "task": { "tasktypeid": 0, # "0" for backtesting "strategyid": strategy["strategyid"], "isbenchmark": 0 } } resp = requests.post("https://api.tradematic.com/taskmanager/tasks", data = json.dumps(task), headers = {"X-API-KEY":apikey}) taskid = json.loads(resp.content)["taskid"] # Sleep for 1 minute time.Sleep(60) # Let's get results of our task resp = requests.get("https://api.tradematic.com/taskmanager/tasks/{0}/result2", headers = {"X-API-KEY":apikey}) result = json.loads(resp.content) # Get the statistical results of backtesting APR = result["apr"]; drawdown = result["drawdown"] # Get the data for the strategy equity curve resp = requests.get("https://api.tradematic.com/taskmanager/tasks/{0}/equity", headers = {"X-API-KEY":apikey}) equitycurve = json.loads(resp.content) for equity in equitycurve: print("Date: {0} Equity: {1}".format(equity["timestamp"], equity["equity"]))
-
Launch strategy automatic execution
import requests import json # Replace with the correct API key apikey = "YOUR_API_KEY" # Let's take the first of our trading accounts resp = requests.get("https://api.tradematic.com/cloud/accounts", headers = {"X-API-KEY":apikey}) account = json.loads(resp.content)[0] # Now start automatic execution of the "SMA-9" strategy on the account "account" startparams = { "data": { "strategyname": "SMA-9", "accountid": account["accountid"] } } requests.post("https://api.tradematic.com/cloud/strategies/start", data = json.dumps(startparams), headers = {"X-API-KEY":apikey})
-
Get account equity chart
import requests import json # Replace with the correct API key apikey = "YOUR_API_KEY" # Make a REST API call resp = requests.get("https://api.tradematic.com/cloud/accounts", headers = {"X-API-KEY":apikey}) # Let's take the first of our trading accounts account = json.loads(resp.content)[0] # Get the account snaphshots data resp = requests.get("https://api.tradematic.com/cloud/accounts/{0}/snapshots".format(account["accountid"]), headers = {"X-API-KEY":apikey}) snapshots = json.loads(resp.content) # Let's show them for snapshot in snapshots: print("Date: {0} Value: {1}".format(snapshot["timestamp"], snapshot["value"]))
Pricing
A user is an account owner that has interacted with your Tradematic-Cloud-based app during the month.
About Us
Tradematic Cloud was created by the team of Tradematic.com.
Since 2009, we've been developing software for automated trading and asset management on stock, futures, forex and cryptocurrency exchanges.
Based on our experience in software development projects for investment institutions and retail traders, we have standardized our best solutions in order to share them with the world.
If you have any questions, please contact us at support@tradematic.com.
Start using Tradematic Cloud immediately and for free.
After signing up, you will receive your API key and a link to API sandbox.